I plan on having an iPhone Screen and an iPad screen with the one module supplying methods for both. My thought was I could pass the view like this.
In View I call Method1(self)
In Modual Method1(lview as iosView)
lview.TableName.RemoveAll
however apparently I can’t do this because I get a compiler error lview has no member named TableName
Whats the best way to do this.
You can’t do it like that because lview As iOSView is just a generic iOSView and has no knowledge of your specific view with the TableName iOSTable on it.
Here are two suggestions:
- In Method1, check the type and cast:
If lView IsA iPhoneView Then
iPhoneView(lView).TableName.RemoveAll
ElseIf lView IsA iPadView Then
iPadView(lView).TableName.RemoveAll
End If
- Pass the iOSTable instead of the entire View:
Method1(table As iOSTable)
table.RemoveAll
It may make more sense to use auto-layout and just have the one view used for both iPad and iPhone, but if you absolutely need to do it that way, you’ll have to specify/typecast the view. Your parameter of iOSview is too generic, and the basic iOS view doesn’t have a table built into it.
Do something like this in the module’s method to point to the table and use it through your method::
[code]dim theTable as iOSTable
if lview isa YouriPadViewClass then
theTable = YouriPadViewClass(lview).TableName
elseif lview isa YouiPhoneViewClass then
theTable = YouriPhoneViewClass(lview).TableName
else
return
end if
theTable.RemoveAll
theTable.AddSection
…etc[/code]
If you do it with auto layout how would you do it?
It would be nice if you could have two sets of constraints, one for iPad and one for iPhone.
Is there a way to do that dynamically?
It depends on what you’re trying to do with the view in terms of layout.
You can name your constraints in the auto-layout editor, and then in the open event of the view check if it’s iPad/iPhone and modify the constraints based on that. It all depends on if that would be more work for you than to keep two separate views with shared methods in a separate module.
Here’s how:
-
Name your constraints like so:
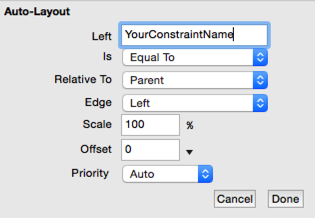
-
In the open event check if it’s an iPad/iPhone, here’s one declare that seems to work fine for that:
[code]Function DeviceType() As text
declare function NSClassFromString lib “Foundation” (clsName as cfstringref) as ptr
declare function currentDevice lib “UIKit” selector “currentDevice” (clsRef as ptr) as ptr
dim currentDeviceRef as ptr = currentDevice(NSClassFromString(“UIDevice”))
declare function deviceName lib “UIKit” selector “model” (classRef as Ptr) as CFStringRef
dim t as text
dim c as CFStringRef
c = deviceName(currentDeviceRef)
t = c
if t.IndexOf(“iPad”)<>-1 then
Return “iPad”
else
Return “iPhone”
end if
End Function
[/code]
- Then based on iPhone/iPad, change the constraints using code like this:
[code]dim forIPad as boolean = DeviceType = “iPad”
Dim c As iOSLayoutConstraint = Self.Constraint(“YourConstraintName”)
if c<>nil then
if foriPad then
c.Offset = 100
else
c.Offset = 50
end if
end if[/code]
Thank you, this helps a lot.